In this article, we’ll take a look at how to setup our Django project to utilize environment variables in Python. Hence, our Django application can use environment variables to retrieve sensitive information that it requires for correct operation such as SECRET_KEY.
Simply explained, environment variables are a collection of dynamically named values that are kept in the system and utilized by applications. You may use these variables to change how individual apps and services interact with the system. Each variable has a name and a value associated with it. Usually, the name is in UPPER CASE, and the values are, of course, case-sensitive.
It’s crucial to keep critical code, such as API keys and passwords, out of the hands of inquisitive eyes. It’s better not to put them on GitHub! Securing your environment variables will create good habits and prevent bothersome emails from GitGuardian, even if you’re working on a personal project with no real users.
How to setup Environment Variable In Django?
Install Django Environ Package
In your terminal, inside the project directory, type:
pip install django-environ
Import environ in settings.py
import environ
Initialise environ
In my Django application I’d like to access environment variables in my settings.py file. Hence, in my settings.py file I’ll do the following.
import environ
# Initialise environment variables
env = environ.Env()
environ.Env.read_env()
Create your .env file
Create a file named ‘.env’ in the same directory as settings.py.
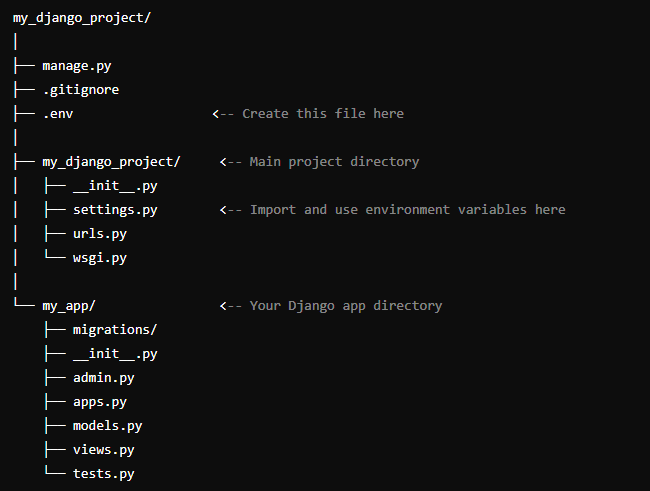
Declare your environment variables in .env
NOTE: Make sure you don’t use quotations around strings.
SECRET_KEY=h^z13$qr_s_wd65@gnj7a=xs7t05$w7q8!x_8zsld#
DATABASE_NAME=postgresdatabase
DATABASE_USER=alice
DATABASE_PASS=supersecretpassword
IMPORTANT: Add your .env file to .gitignore
Create a.gitignore file at the project root if you don’t already have one.
Make sure your.env file’s name is included.
If you’re not sure what other file types should be in the.gitignore, have a look at this example.
Utilize the env() function to access environment variable
I’d like to access the DATABASE_NAME, DATABASE_USER, and DATABASE_PASS enviroment variables in my settings.py file for my Django app. Hence, I use the env() function to achieve this result. The env() function comes from the environ package we installed, and you should have had initialised the environ package already. As I did in my previous steps for our settings.py file.
DATABASES = {
‘default’: {
‘ENGINE’: ‘django.db.backends.postgresql_psycopg2’,
‘NAME’: env(‘DATABASE_NAME’),
‘USER’: env(‘DATABASE_USER’),
‘PASSWORD’: env(‘DATABASE_PASS’),
}
}
And also in settings.py
SECRET_KEY = env(‘SECRET_KEY’)
That is it you’r done.
Conclusion
In this article, we took a look at how to work with environment variables in your Django app. Now you should feel at ease dealing with scenarios where you’r required to use environment variables with your Django app, such as the settings.py file. As always, If you have found this article useful do not forget to share it and leave a comment if you have any questions.
Happy Coding…!!!
Leave a Reply