In today’s tutorial, I’m going to show you how to create custom splash screen for your Flutter application. By the end of this tutorial, you’ll understand how to create a custom splash screen that transitions smoothly into your application. Let’s dive in!
Overview
We’ll create a custom splash screen using the flutter_native_splash
package, which simplifies the process by generating the necessary settings and files for both iOS and Android. Our splash screen will display an image and a background color before transitioning to the main application.
Prerequisites
Before starting, ensure you have:
- Flutter installed
- Basic knowledge of Flutter and Dart
Setting Up the Project
Step 1: Add Dependencies
First, we need to add the flutter_native_splash
dependency to our project. Open the pubspec.yaml
file and add the following line under dependencies:
dependencies:
flutter:
sdk: flutter
flutter_native_splash: ^1.2.3
To gwt thw dependency click here.
This package will help us configure and generate the necessary splash screen settings for both Android and iOS platforms.
After adding the dependency, run flutter pub get
to install it.
flutter pub get
Step 2: Configure Splash Screen
Next, we need to configure the splash screen settings in the pubspec.yaml
file. Add the following configuration:
flutter_native_splash:
color: "#42a5f5"
image: assets/images/splash/logo.png
android: true
ios: true
web: true
android_12:
color: "#42a5f5"
image: assets/images/splash/logo.png
This configuration specifies the background color and the image for the splash screen. It also ensures that the splash screen is generated for Android, iOS, and web platforms.
Main Application File
Now, let’s set up our main application file. This file will initialize the splash screen and configure the Flutter app.
Importing Necessary Packages
Start by importing the necessary packages in main.dart
:
import 'package:flutter/material.dart';
import 'package:flutter_native_splash/flutter_native_splash.dart';
These imports are essential for setting up the splash screen and running the Flutter application.
Initializing the Splash Screen
In the main
function, we need to initialize the splash screen and configure the Flutter app to display it:
void main() async {
WidgetsBinding widgetsBinding = WidgetsFlutterBinding.ensureInitialized();
FlutterNativeSplash.preserve(widgetsBinding: widgetsBinding);
await Future.delayed(
Duration(seconds: 5),
);
FlutterNativeSplash.remove();
runApp(const MyApp());
}
Here’s what each part does:
WidgetsBinding widgetsBinding = WidgetsFlutterBinding.ensureInitialized();
: Ensures that the Flutter framework is properly initialized.FlutterNativeSplash.preserve(widgetsBinding: widgetsBinding);
: Preserves the splash screen until we manually remove it.await Future.delayed(Duration(seconds: 5));
: Delays the removal of the splash screen for 5 seconds.FlutterNativeSplash.remove();
: Removes the splash screen after the delay.runApp(const MyApp());
: Runs the main application.
Creating the MyApp Widget
Next, we create the main application widget:
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const HomePage(),
);
}
}
MyApp
is a stateless widget that returns aMaterialApp
.MaterialApp
configures the app’s title, theme, and home page.
Creating the HomePage Widget
Finally, we create the home page widget:
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return const Scaffold(
body: Center(
child: Text("Howdy!"),
),
);
}
}
HomePage
is a stateless widget that returns aScaffold
with a centered text saying “Howdy!”.
Adding the Splash Screen Image
To display the splash screen image, create the required folder structure and add your splash screen image:
assets/
└── images/
└── splash/
└── logo.png
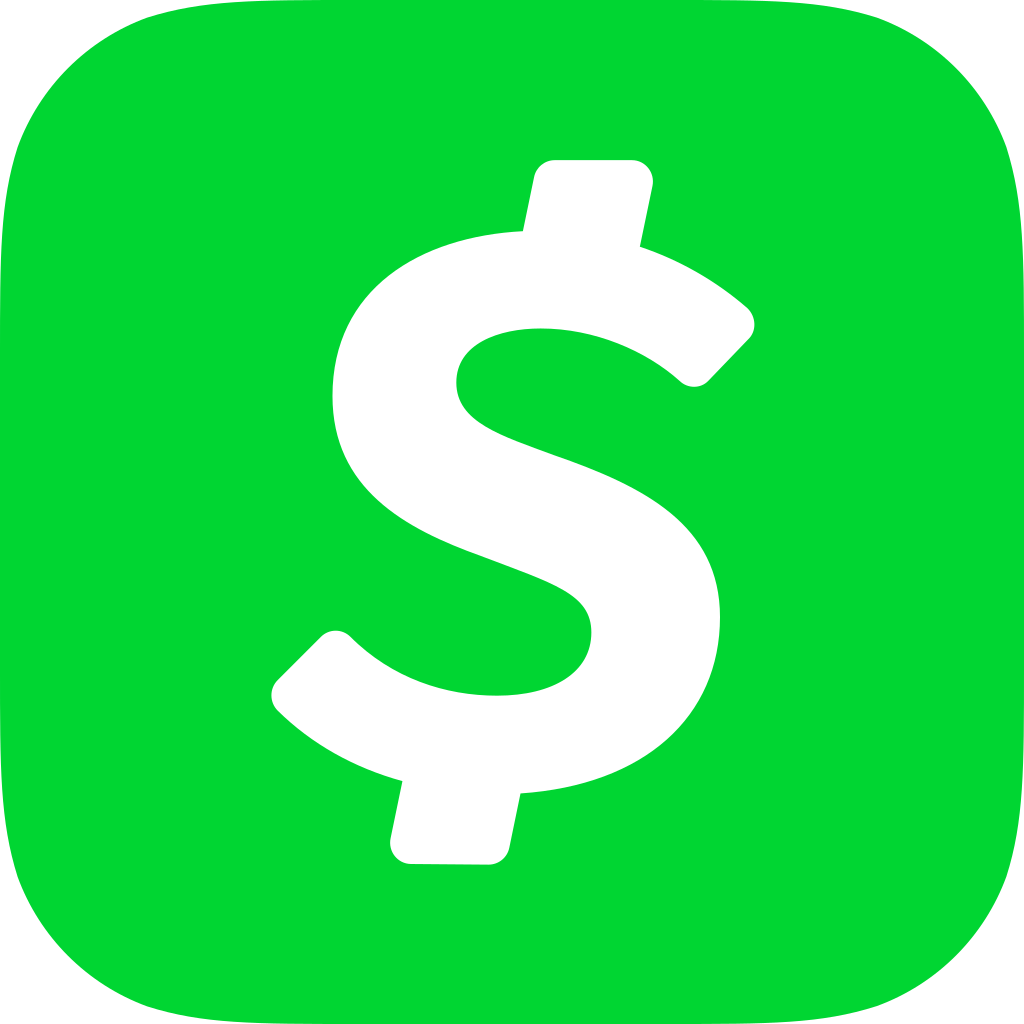
Update the pubspec.yaml
file to include the assets:
flutter:
assets:
- assets/images/splash/logo.png
Running the Application
With everything set up, run your application using flutter run
. You should see a splash screen with your image and background color for 5 seconds before transitioning to the main application screen.
flutter run
Customizing for Android 12 and Above
For devices running Android 12 and above, additional settings are required. Ensure the android_12
section in your pubspec.yaml
file includes the color and image properties:
android_12:
color: "#42a5f5"
image: assets/images/splash/logo.png
Get Source Code for free:
Conclusion
In this tutorial, we built a custom splash screen for a Flutter application using the flutter_native_splash
package. This package simplifies the process of creating splash screens for both iOS and Android, ensuring a smooth transition into the main application.
If you have any questions, comments, or concerns, feel free to leave them in the comments section below, and I’ll do my best to address them. Stay happy, stay healthy, keep learning, and keep growing. See you in the next blog!
Leave a Reply